Introduction
Welcome, developers! Today, we’re going to cover a significant feature of Empress: QUnit Testing. This feature is a powerful tool for writing UI tests using the QUnit framework and native frappe API. It allows developers to write tests directly in JavaScript, providing a faster way of developing tests.
Understanding QUnit Testing
QUnit is a powerful, easy-to-use JavaScript unit testing framework. It’s used by the jQuery, jQuery UI, and jQuery Mobile projects and is capable of testing any generic JavaScript code.
Test Runner
Empress utilizes a Test Runner to facilitate QUnit-based testing. To write tests, you simply add your test files to the tests/ui
folder of your application. These files must begin with test_
and end with a .js
extension.
The Test Runner provides a user interface to load all your QUnit tests and run them in the browser. In the CI, all QUnit tests are run by the Test Runner using frappe/tests/test_test_runner.py
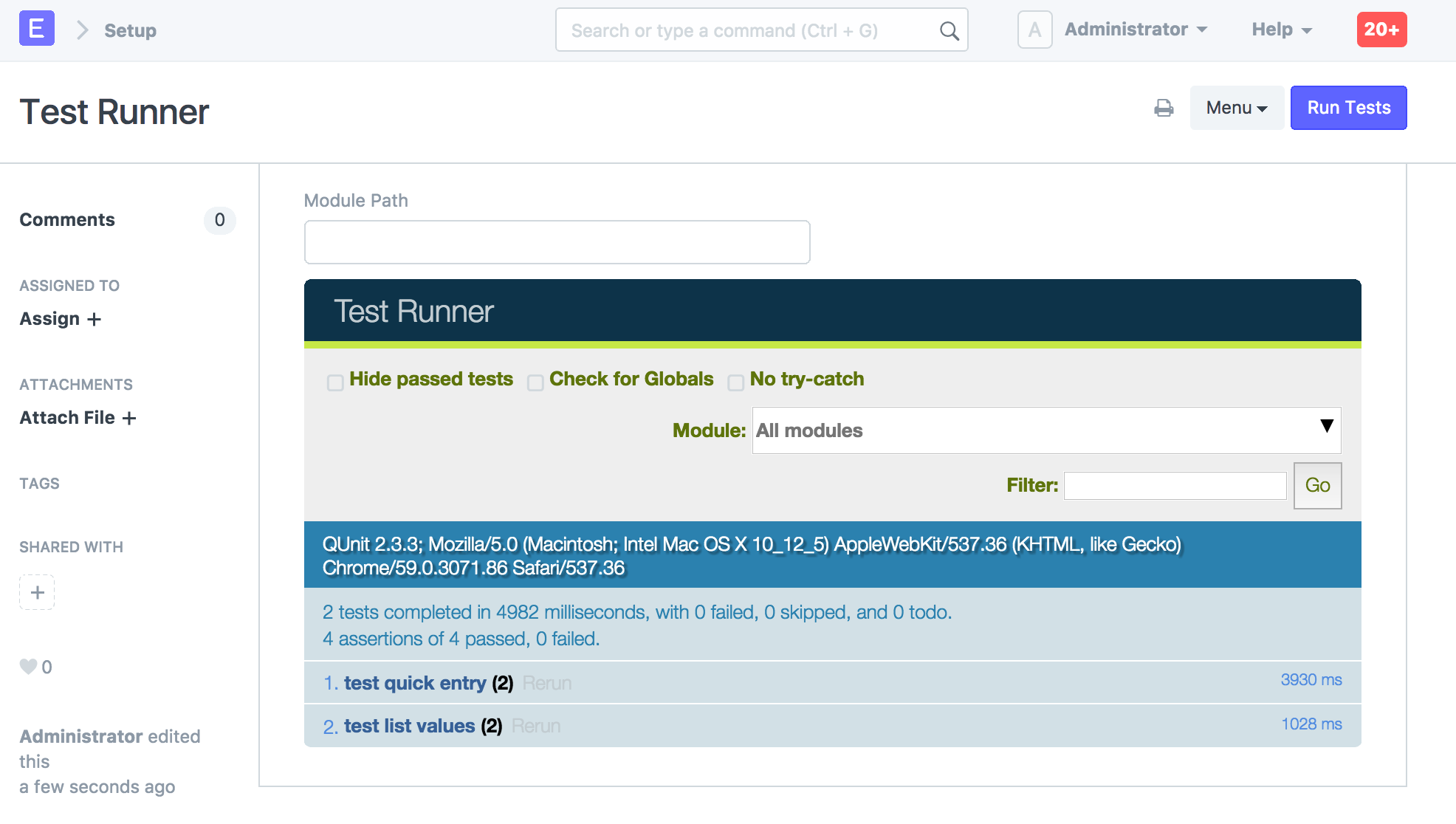
Running Tests
To run a Test Runner-based test, use the run-ui-tests
bench command, passing the name of the file you want to run.
bench run-ui-tests --test frappe/tests/ui/test_list.js
This command passes the filename to test_test_runner.py
, which loads the required JS in the browser and executes the tests.
Debugging Tests
To debug a test, you can open it in the Test Runner from your UI and run it manually. This allows you to see exactly where the test is failing.
Test Sequence
In Empress, UI tests are run in a fixed sequence to ensure dependencies. The sequence in which the tests will be run is defined in the tests/ui/tests.txt
file.
Running All UI Tests
To run all UI tests for your app, use the following command:
bench run-ui-tests --app [app_name]
This command runs all the files in your tests/ui
folder one by one.
Example QUnit Test
Here’s an example of a To Do test in QUnit:
QUnit.test("Test quick entry", function(assert) {
assert.expect(2);
let done = assert.async();
let random_text = frappe.utils.get_random(10);
frappe.run_serially([
() => frappe.set_route('List', 'ToDo'),
() => frappe.new_doc('ToDo'),
() => frappe.quick_entry.dialog.set_value('description', random_text),
() => frappe.quick_entry.insert(),
(doc) => {
assert.ok(doc && !doc.__islocal);
return frappe.set_route('Form', 'ToDo', doc.name);
},
() => assert.ok(cur_frm.doc.description.includes(random_text)),
// Delete the created ToDo
() => frappe.tests.click_page_head_item('Menu'),
() => frappe.tests.click_dropdown_item('Delete'),
() => frappe.tests.click_page_head_item('Yes'),
() => done()
]);
});
Writing Test Friendly Code with Promises
Promises are a crucial aspect of writing test-friendly code. If your method calls an asynchronous call (ajax), then you should return a Promise
object. If you encounter a function that does not return a Promise
object while writing tests, you should update the code to return a Promise
object.
Conclusion
In conclusion, QUnit Testing in Empress is a powerful feature that allows developers to write, run, and debug tests directly in JavaScript. It ensures reliable and efficient testing, reinforcing the robustness and quality of the software. With its easy integration and utilization, it greatly contributes to the development and customization of business solutions.